Hello friends, I got a quick question. I was looking at a nice dev update over at ThinMatrix and noticed he's making his own UI system:
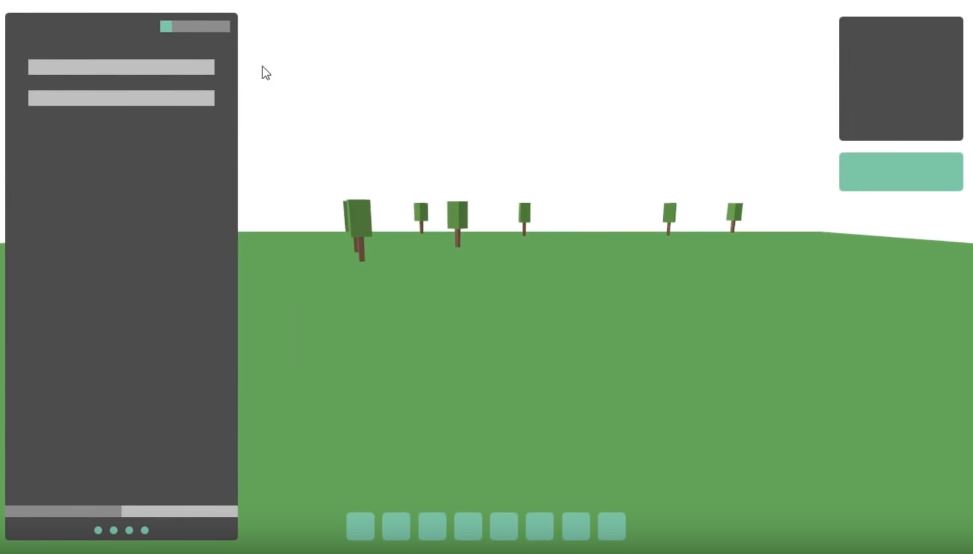
Question about UI Elements:
Sounds like a nice project. What would be the best way to implement these windows? Because I could just render quads on the screen, but wouldn't that be a single draw call per each element?
I know how instancing works now, so I'm guessing I'd have to instance all the UI elements so all UI elements represent one draw call, am I right about that?
--------------------------------------------------------------------------
Question about Font Rendering:
My question regarding fonts is very similar. I noticed reading tutorials I have to make a “spritesheet” of all the font's available letters and update quads on screen with those letters (as textures).
My question is, do I also have to batch these quads so it's not a draw call per letter? What's the current go to way to render text in an OpenGL game?
Thanks again everybody, always helpful!