Now that my school projects have been handed in and all my exams are behind me I will hopefully be able to spend more time developing arkanong.
Let's take a look at how we can make the gameballs bounce off each other.
There are three important things to keep in mind about our game physics:
a) all balls have the same mass, meaning they all "weigh" the same.
b) all collisions are perfectly elastic, so no energy will be converted to another form.
This means that after two balls bounce off each other they will retain their kinetic energy (in real life part of it would be converted to sound waves, heat, etcetera).
c) all movements/collisions are frictionless
In a real pool game the friction of the felt would cause the ball to come to a halt after a while. We want our gameballs to keep moving indefinitely so
our physics will be completely frictionless
These three properties enable us to greatly simplify our physics formulas, as you will see further on.
The sphere to sphere collision detection/response consists of the following steps:
1) detect a collision between two balls.
This step is pretty easy; we simply check if the distance between two balls is less than (or equal to) the sum of their radiuses.
The meaning of collision normal will be explained in step two, for now just think of it as the line that connects the two centres of the ball.
The distance between the two balls is calculated using the pythagorean theorem.
float radius1 = ball1->getSprite().getLocalBounds().width / 2;float radius2 = ball2->getSprite().getLocalBounds().width / 2;//minimumDistance for collision = radius1 + radius2float minimumDistance = radius1 + radius2;//amount of pixels between the centresfloat actualDistance = sqrt((collisionNormal.x * collisionNormal.x) + (collisionNormal.y * collisionNormal.y));//if the distance <= radius1+radius2 a collision has occuredif (actualDistance <= minimumDistance){ [collision response code goes here]}
2) determine the collision normal.
The collision normal is the unit vector that points in the direction of the collision (i.e. the vector that points toward the place where the two balls are touching). To calculate this vector we simply connect the centres of the two balls. We already did this in the first step to determine if the gameballs are touching. All that remains to be done is to turn it into a unit vector (that is: make the vector have a magnitude of 1).
//normalize collision vectorcollisionNormal.x /= actualDistance;collisionNormal.y /= actualDistance;
3) determine the unit tangent.
Besides the collision normal we will also need the vector which is perpendicular to it, a.k.a. the tangent vector.
Because we already have the collision normal it is very easy to calculate the unit tangent: we simply "flip" the collision normal and make y negative.
sf::Vector2f unitTangent(-collisionNormal.y, collisionNormal.x);
try this out on a piece of paper, it really works!
4) project the velocity of the ball onto the unit tangent & unit normal vectors.
Projecting the velocity vector on the tangent & normal will decompose it into two components. You could think of them as x and y - in reality it's the part that is affected by the collision (= normal component), and the part that isn't (= tangent component).
Think of a ball that is moving horizontally from left to right. When another ball grazes it on the bottom it will no longer be moving horizontally, but it will still be moving from left to right. Because the tangent and normal are unit vectors, we can project the velocity vectors onto them by calculating the dot product. (this is vector math)
//----------project velocities onto unit normal & unit tangent vector-----//this will decompose the velocities into a normal and a tangent componentsf::Vector2f ball1Velocity = ball1->getVelocities();double ball1NormalComponent = (collisionNormal.x * ball1Velocity.x) + (collisionNormal.y * ball1Velocity.y);double ball1TangentComponent = (unitTangent.x * ball1Velocity.x) + (unitTangent.y * ball1Velocity.y);sf::Vector2f ball2Velocity = ball2->getVelocities();double ball2NormalComponent = (collisionNormal.x * ball2Velocity.x) + (collisionNormal.y * ball2Velocity.y);double ball2TangentComponent = (unitTangent.x * ball2Velocity.x) + (unitTangent.y * ball2Velocity.y);
5) Adjust the normal components of the ball velocities.
As stated in the previous step, the velocity has a component that isn't affected (= tangent) and a component that is (= collision normal). In this step, we adjust the part of the velocity vector that will react to the collision, in other words: this is where the collision response is actually occuring.
In Newtonian physics the adjusted normal velocities of two colliding spheres is calculated with the following formulas:
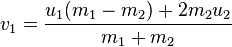

where V is the new normal velocity after the collision, U is the old normal velocity before the collision, and M is the mass of the ball.
Remember when I said that all balls "weigh" the same? This is where that knowledge comes in handy for reducing the complexity of the physics. Substitute m1 and m2 with the number 1 (or any number for that matter) and see what happens:
V1 = (U1 * 0) + (2/2) * U2
V1 = 0 + 1 * U2
V1 = U2
you can just switch the two normal components!
//-----------------switch normal components---------------//mass is the same for all balls, so the collision response function can be greatly simplified.//we simply switch the components.double newBall1Component = ball2NormalComponent;double newBall2Component = ball1NormalComponent;
6) multiply the components with the two unit vectors.
By calculating the two components (normal & tangent) we now have the amount of pixels by which the ball should shift along these directions
(you could think of this as "5 pixels
and 10 pixels [up]", but again it's really the part of the velocity that is and isn't affected by the collision).
These two numbers correspond to the magnitude of the new velocity vectors ("5 pixels and 10 pixels"), but we still need convert them into actual vectors via multiplication. (that is, give them a direction or make it "5 pixels to the left and 10 pixels up"). Actually we only need the normal part, because the tangent component is unaffected by the collision. I included the calculation anyway.//---------------convert components back into vectors----------sf::Vector2f newBall1NormalVector(0.0f, 0.0f);newBall1NormalVector.x = newBall1Component * collisionNormal.x;newBall1NormalVector.y = newBall1Component * collisionNormal.y;//these actually remain the same as before but we leave the calculation in for claritysf::Vector2f newBall1TangentVector(0.0f, 0.0f);newBall1TangentVector.x = ball1TangentComponent * unitTangent.x;newBall1TangentVector.y = ball1TangentComponent * unitTangent.y;sf::Vector2f newBall2NormalVector(0.0f, 0.0f);newBall2NormalVector.x = newBall2Component * collisionNormal.x;newBall2NormalVector.y = newBall2Component * collisionNormal.y;//these actually remain the same as before but we leave the calculation in for claritysf::Vector2f newBall2TangentVector(0.0f, 0.0f);newBall2TangentVector.x = ball2TangentComponent * unitTangent.x;newBall2TangentVector.y = ball2TangentComponent * unitTangent.y;
7) add the components back together.
Earlier we decomposed the velocity of the balls into two components (normal & tangent), now that we've recalculated these two components we simply add them back together to get the new velocity for the ball. ('5 to the left and 10 up' becomes '14 diagonally')sf::Vector2f newBall1Velocity = newBall1TangentVector + newBall1NormalVector;sf::Vector2f newBall2Velocity = newBall2TangentVector + newBall2NormalVector;
Now, because I store angles rather than velocity vectors I do some additional trigonometry on these but if you work with vectors in your code then you're as good as done, all that needs doing is setting the new directions for the balls.
I realize this is probably confusing as hell if you're not familiar with physics or vector mathematics. Check out these links if you want to get a better grasp of what's going on here - sooner or later every game programmer is going to come into contact with these things so it's better to start early.
http://en.wikipedia.org/wiki/Elastic_collision
http://www.vobarian.com/collisions/2dcollisions2.pdf
http://nicoschertler.wordpress.com/2013/10/07/elastic-collision-of-circles-and-spheres/
As always you can check out the code for the game on github.
The code shown in this article can be found in source -> ObjectManager.cpp -> performCircleToCirlceCollisionChecks()