After a short break and attending GDC I am back to making a post. Work on The Krilling has been minimal, so instead today I am going to talk about a side project of mine in the works. This past month and a half I have been working on learning Godot. Today I am going to talk about the beginning of that journey specifically the process of recreating one of my unity projects in Godot. I wrote a post about the original project previously that you can read here.
To start off I through a rigid body in the scene and got to work programming. My first goal was to simply create a dash functionality that allows the player to dash in the direction of the cursor. To do so I first set up an InputEvent in the InputMap to keep track of left-click presses. Then once that event is triggered I start the dash. To achieve the dash I get the direction that was clicked by subtracting the mouse position from the position of the player and normalizing it. Then it is as simple as applying an impulse to the rigid body in that direction, done. Well, if you read my last post then you might remember that there is one additional step. Say the player is traveling at a high velocity to the right and attempts to dash to the left. With this, the dash would only lower the speed to the right rather than sending the player to the left.
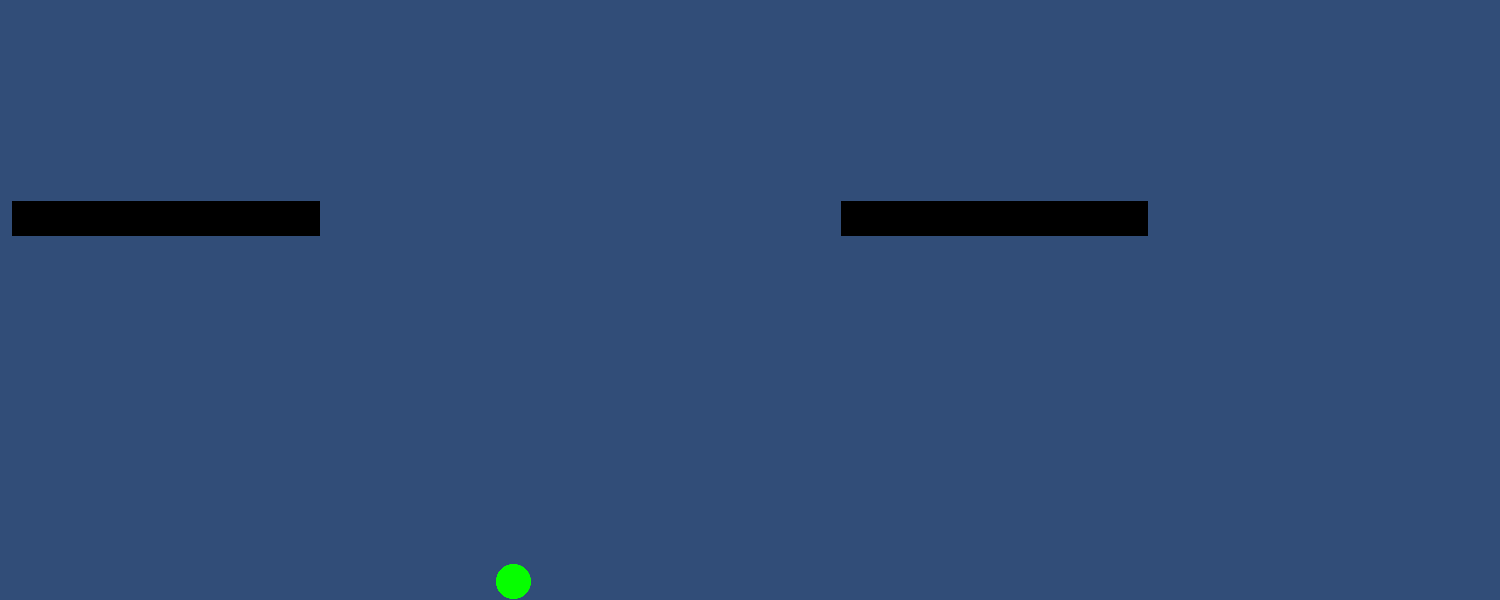
Here is an example gif of this from my previous post.
To stop this from happening we compare the player's current velocity to the target dash velocity. If the velocities are in opposite directions on either the x or the y-axis than that part of the velocity is subtracted from the target velocity. This has the effect of when applying a force of -1 on the x-axis to a player that currently has a velocity of 1, we end up adding a force of -2, which cancels out the 1 and gets our desired velocity of -1.
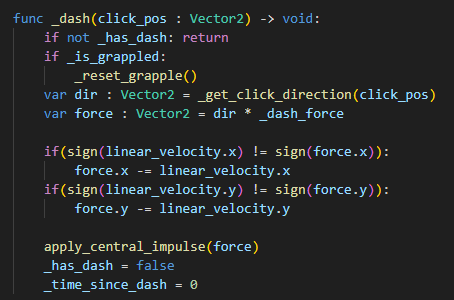
The next step was then to recreate the grappling hook which was the majority of the work last time. However, this time it should be easy since the logic is already there. First I need to recreate the base functionality of shooting the hook and having it collide. To do so I, like before, have an emitter object on the player that handles shooting the hook. Like the dash, I have an InputEvent set up for right-click to trigger the shooting of the hook.
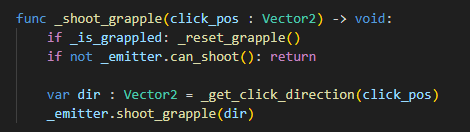
Once the hook is shot the emitter increases the current end position of the hook over time and updates the visual to match this position. To do so I set the tip position to the end position and I modify the scale of the line to fit between the player and the end position and rotate it to face the correct direction. Then to stop the hook from going to infinity I destroy it if its length exceeds some arbitrary value.
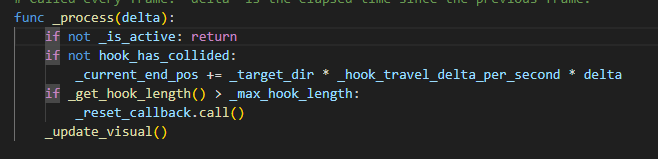

I then have an Area2D on the tip of the hook that emits a signal when it enters something. In that case, the hook has hit and that information is provided back to the player script through a callback function (realistically should be a custom signal, but at the time of writing this code I did not know about that).
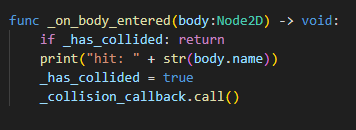
Now we have a working grappling hook and it's time to create the swinging aspect of this. For more details, you can check out my previous post, but the crux of it is to convert the player velocity to be in the hook's local space so that the velocity is described in terms of the direction of the hook and perpendicular to the direction of the hook. Then if the axis that is going in the direction of the hook has a velocity traveling away from the hook, we instead set it to some arbitrary constant representing the return speed. This allows us to clamp the player to not travel away from the hook, but also allow them to retain their velocity in any other direction. Ignore the actual transformation of velocity for now.
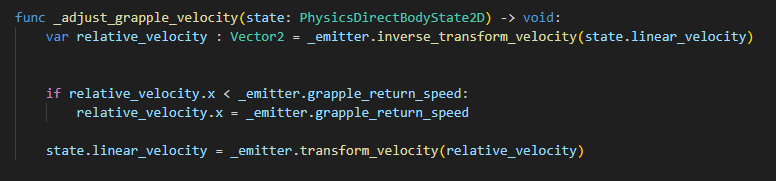
Now the hard part becomes how exactly do we do this. In Unity, this was fairly trivial because it had built-in transform direction and inverse transform direction functions, but Godot does not. However, it does have a transform point and inverse transform point in the form of to_global, and to_local. So all we have to do is describe the direction as a point and use these. So to transform global velocity to the local space of the hook we simply take our velocity, (which can be interpreted as a point relative to the origin) and add the global position of the hook (to create a point that is the original velocity distance away from the hook) and call to_local to convert it to local space. Then to do the reverse we take the local velocity and use to_global to convert it to a global point, which then gives us a point the global velocity away from the hook position. Then we just have to subtract the global position to get a point the velocity away from the origin, aka the global velocity we were looking for.

That is going to be all for now. I have a lot more done on this project, so stay tuned for future logs where I go over that in addition to any Krilling updates.